Today the team at Metafold 3D is thrilled to announce the release of version 0.1 of the Metafold Software Development Kit (SDK) for Python. The SDK is entirely open source, visit the GitHub repo to get started.
The Metafold SDK for Python, like many other web service SDKs, is a small Python package that aims to make interacting with the Metafold REST API feel more natural. The SDK is aimed at technical users: designers, researchers, and developers alike—that are familiar with Python programming and would like to automate implicit modeling or geometry processing workflows for both general CAD purposes and additive manufacturing (AM). This release forms the foundation for what we’re hoping will be a suite of software components that will enable our users to innovate more with implicit shapes, read to the end to find out more!
REST API
The Metafold REST API has been available to all license tiers since the beginning of 2024 (you may find the documentation for it here), but until now we haven’t written much about how users may find it useful. Some of our users have had success in directly using the API to carry out the research of novel lattice metamaterials and leveraging the asynchronous job system to build their own web applications. We hope the SDK and its future developments will enable more users to access API functionality in order to address their business needs.
STL mesh export
Diving straight in, let’s see how we can use the SDK to export a STL mesh from an existing project on the Metafold platform. You’ll need your secret access token and the ID of a project you’d like to use—feel free to duplicate this example project to follow along with.
We start by importing and initializing an instance of the Metafold API client which provides access to all of the API endpoints.
Implicit shape definition
To export a mesh we need to first download the full implicit shape definition, a graph of “operators” encoded as JSON, from our project. Our cloud-based implicit geometry kernel expects this JSON graph as input and produces a variety of outputs—most commonly a raw binary blob containing evaluation results, that is then consumed by other processes to generate last-mile output, e.g. a STL mesh file.
You can export the JSON graph through the Metafold app.
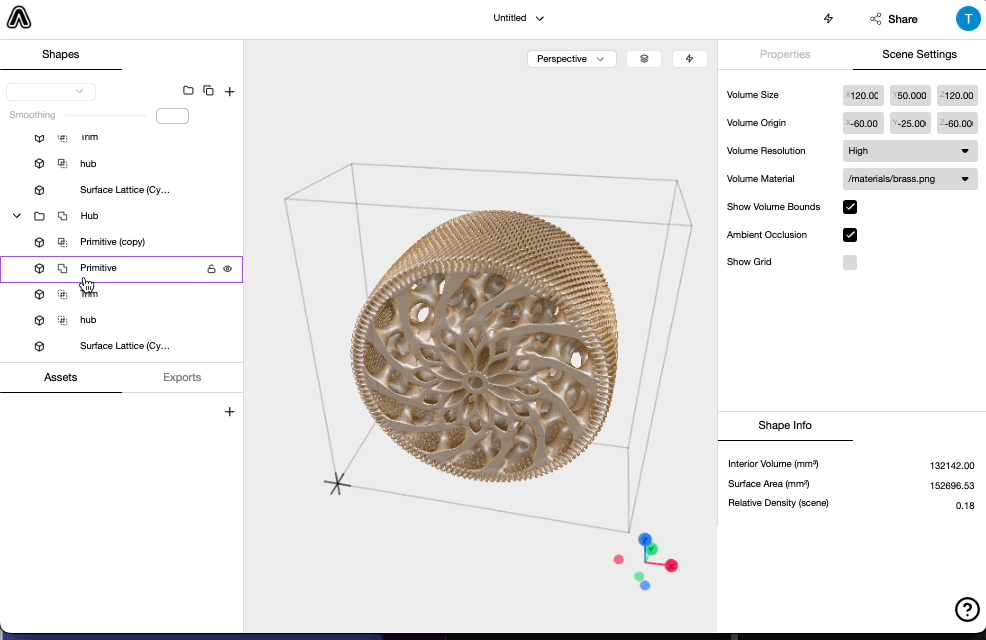
Your graph should look similar to the following (link to full example):
Each graph is composed of a list of operators and a list of edges representing connections between these operators. By convention, graphs exported from the app begin with a GenerateSamplePoints
operator at index 0 and end with a Threshold
operator. The presence of these operators is required for many of the jobs that our API provides.
Running an export job
In this example we run an Export Triangle Mesh job to evaluate the implicit shape and generate a STL mesh asset. The parameters required by each job are described in the API docs, in particular note the point_source
parameter for Export Triangle Mesh, which expects the index of the GenerateSamplePoints
operator we mentioned earlier.
All of the jobs provided by the Metafold API run asynchronously. metafold.jobs.run
handles waiting on the job’s progress until its completion. The returned Job
is a resource that contains the original request parameters alongside any generated assets and metadata, here is a type-annotated, simplified version of the Job
class:
The Export Triangle Mesh job produces a single asset, which we can download using its ID.
Depending on the complexity of your implicit shape the generated file may be quite large (the medium resultion export for the example project resulted in a 583 MB STL file).
The asynchronous job system is where most of the utility of the Metafold REST API is found. Jobs consume input parameters and assets, and produce more assets (and occasionally some useful metadata). We have a growing library of jobs that fulfill a wide variety of needs, from:
- exporting a tessellated mesh from an implicit shape, as demonstrated in this example; to
- slicing an implicit shape for AM (as a PNG stack or CLI file); to
- computing the surface area and interior volume of an implicit shape.
Asset management
Many of our jobs require input assets, and most of them produce at least one asset as output. For the most part when we talk about an asset, we’re referring to a file that has been uploaded by the user or generated by a job, and is being managed by our API. The SDK includes a handful of methods for managing assets.
Filter queries use a simple syntax and are highly recommended to reduce the size of the response and consequently improve response times. For example to list all STL files in a project:
For more on the query syntax please refer to the API docs.
The examples we’ve covered in this blog post only scratch the surface of what you can do with the SDK. You can explore some other complete examples in the GitHub repo.
Future plans
As the SDK develops we will be introducing functionality to help users build shape definitions programmatically, with tools to validate operator connections, and higher-level building blocks that encapsulate useful/common patterns we’ve identified while building graphs ourselves internally.
We look forward to seeing how the SDK will help you supercharge your AM workflows. Please feel free to ask questions and raise issues on GitHub.
Don’t miss the release of the Metafold SDK for Javascript in the coming weeks, watch this space for updates!